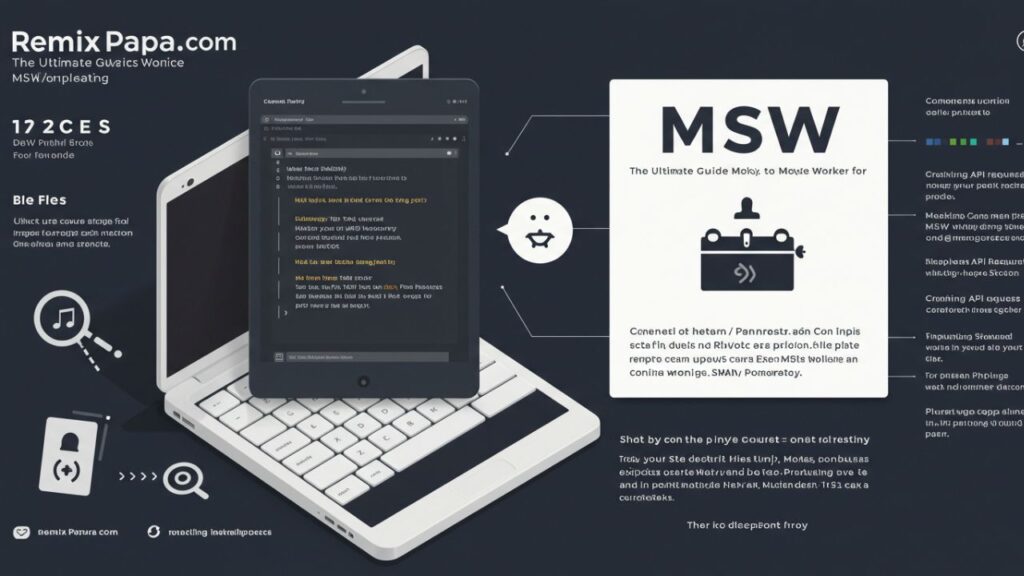
RemixPapa.com MSW
RemixPapa.com MSW: The Ultimate Guide to Mock Service Worker for Remix Applications
The RemixPapa.com MSW ecosystem offers developers a powerful approach to testing Remix applications with realistic API mocking. Mock Service Worker (MSW) transforms how frontend teams handle API dependencies during development and testing phases. Developers absolutely need this tool to create reliable, maintainable applications that function perfectly even when backend services become unavailable.
Introduction to RemixPapa.com MSW
RemixPapa.com MSW combines the robust capabilities of Mock Service Worker with the innovative Remix framework to create a seamless development experience. Teams constantly struggle with reliable API testing during development, which often leads to bugs in production. Additionally, frontend developers frequently wait for backend teams to complete APIs before they can begin implementation.
Furthermore, the challenges multiply when developers try to test edge cases or error states that rarely occur in production environments. MSW solves these problems elegantly by intercepting network requests at the service worker level rather than changing your application code. Consequently, this approach provides a realistic testing environment without compromising code integrity.
Understanding Mock Service Worker Fundamentals
Mock Service Worker operates on an entirely different principle compared to traditional API mocking libraries. Traditional libraries typically replace the fetch implementation or override specific modules, which creates an artificial testing environment. In contrast, MSW intercepts actual network requests at the browser’s service worker level or Node.js environment.
Moreover, MSW allows developers to define request handlers that determine how the intercepted requests should respond. You can easily simulate success responses, network failures, validation errors, or any other scenario without changing a single line of application code. Therefore, your tests will more accurately reflect real-world usage patterns than ever before.
Why RemixPapa.com MSW Matters for Remix Applications
Remix applications rely heavily on loader and action functions that fetch data from external sources or handle form submissions. Testing these functions thoroughly becomes absolutely critical for maintaining application reliability under various conditions. MSW provides the perfect companion for testing these Remix-specific features without complex mocking setups.
Additionally, Remix emphasizes progressive enhancement and resilient user experiences, which perfectly aligns with MSW’s approach to realistic network simulation. Your team can verify application behavior when network conditions deteriorate or servers respond with unexpected errors. Subsequently, this leads to more robust applications that gracefully handle real-world conditions.
Setting Up MSW in Your Remix Project
Installing MSW in your Remix project requires just a few commands to get started with this powerful testing tool. First, open your terminal and run npm install msw --save-dev
or yarn add msw --dev
to add MSW to your development dependencies. Next, create a directory structure to organize your mocks effectively.
Furthermore, you’ll need to set up the service worker for browser testing with the command npx msw init public/
which copies the necessary service worker file to your public directory. After installation, create a mocks directory with separate files for different API endpoints to keep your mock organization clean and maintainable.
// mocks/handlers.js
import { rest } from 'msw'
export const handlers = [
rest.get('/api/users', (req, res, ctx) => {
return res(
ctx.status(200),
ctx.json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
])
)
}),
rest.post('/api/users', (req, res, ctx) => {
return res(
ctx.status(201),
ctx.json({ id: 3, name: 'New User' })
)
})
]
Integrating MSW with Remix Loaders
Remix loaders fetch data before rendering components, making them perfect candidates for MSW integration. You can easily test loaders with realistic API responses by configuring MSW to intercept those specific endpoints. Your loader functions remain untouched while MSW handles the network interception seamlessly behind the scenes.
Moreover, testing different response scenarios becomes straightforward with MSW’s flexible request handlers. You can simulate successful responses with various data structures, error responses with appropriate status codes, or even network timeouts to verify loader error boundaries. Consequently, your application becomes more resilient against unexpected API behaviors.
// app/routes/users.jsx
import { json } from '@remix-run/node';
import { useLoaderData } from '@remix-run/react';
export async function loader() {
const response = await fetch('/api/users');
if (!response.ok) {
throw new Response("Failed to load users", { status: 500 });
}
return json(await response.json());
}
export default function Users() {
const users = useLoaderData();
return (
<div>
<h1>Users</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
Testing Remix Actions with MSW
Remix actions handle form submissions and data mutations, which makes them critical for application functionality. MSW allows precise testing of these actions by intercepting POST, PUT, PATCH, and DELETE requests sent during form submissions. Your tests can verify both successful submissions and appropriate error handling without complex setup requirements.
Furthermore, you can test validation errors, server errors, or network failures to ensure your action error boundaries function correctly. Action testing with MSW creates confidence that forms will behave consistently regardless of backend conditions. Therefore, users will experience fewer disruptions when network conditions fluctuate in the real world.
// Test file for actions
import { render, screen, fireEvent } from '@testing-library/react';
import { rest } from 'msw';
import { setupServer } from 'msw/node';
import NewUserForm from './NewUserForm';
const server = setupServer(
rest.post('/api/users', (req, res, ctx) => {
return res(
ctx.status(201),
ctx.json({ id: 999, name: 'Created User' })
);
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
test('displays success message after form submission', async () => {
render(<NewUserForm />);
fireEvent.change(screen.getByLabelText(/name/i), {
target: { value: 'New Test User' }
});
fireEvent.click(screen.getByRole('button', { name: /create/i }));
expect(await screen.findByText(/user created successfully/i)).toBeInTheDocument();
});
Browser vs. Node.js Setup for MSW
MSW offers two distinct environments for intercepting network requests depending on your testing needs. The browser setup uses service workers to intercept actual network requests during manual testing or browser-based automated tests. Alternatively, the Node.js setup intercepts requests during unit or integration tests running in a Node environment.
Additionally, you might need both setups for comprehensive testing coverage across different testing scenarios. The browser setup provides a more realistic end-to-end testing experience, while the Node.js setup offers faster test execution for frequent runs. Therefore, most projects benefit from implementing both approaches for complete testing coverage.
// Browser setup (for development and E2E testing)
// src/mocks/browser.js
import { setupWorker } from 'msw'
import { handlers } from './handlers'
export const worker = setupWorker(...handlers)
// Node.js setup (for unit/integration tests)
// src/mocks/server.js
import { setupServer } from 'msw/node'
import { handlers } from './handlers'
export const server = setupServer(...handlers)
Advanced Request Handling Techniques
MSW offers sophisticated request handling beyond basic response mocking for complex testing scenarios. You can access request parameters, headers, and body content to create dynamic responses based on the actual request data. This capability allows testing of search functionality, pagination, or filtering features with realistic data interactions.
Furthermore, you can delay responses to test loading states or simulate slow network conditions using the ctx.delay() function. Response chaining also allows testing retry mechanisms by returning different responses for subsequent requests to the same endpoint. Consequently, these advanced techniques create highly realistic testing scenarios for robust application verification.
// Dynamic response based on request parameters
rest.get('/api/users', (req, res, ctx) => {
const searchTerm = req.url.searchParams.get('search')
if (searchTerm) {
return res(
ctx.json(mockUsers.filter(user =>
user.name.toLowerCase().includes(searchTerm.toLowerCase())
))
)
}
// Pagination example
const page = parseInt(req.url.searchParams.get('page') || '1')
const limit = parseInt(req.url.searchParams.get('limit') || '10')
const startIndex = (page - 1) * limit
const endIndex = page * limit
return res(
ctx.json({
users: mockUsers.slice(startIndex, endIndex),
totalPages: Math.ceil(mockUsers.length / limit),
currentPage: page
})
)
})
Creating Realistic Test Scenarios
Creating realistic test scenarios helps identify potential issues before they reach production environments. MSW excels at simulating various network conditions including slow responses, timeouts, and intermittent failures that might affect user experience. Your team can verify loading states, error handling, and recovery mechanisms under these challenging conditions.
Moreover, testing authentication flows becomes straightforward with MSW by simulating different authentication states or token expiration scenarios. You can also test resource access permissions by returning appropriate status codes based on provided authorization headers. Therefore, security-related features receive thorough testing without complex backend configuration.
// Testing authentication flows
rest.post('/api/login', (req, res, ctx) => {
const { username, password } = req.body
if (username === 'testuser' && password === 'password') {
return res(
ctx.status(200),
ctx.json({
token: 'fake-jwt-token',
user: { id: 1, username: 'testuser' }
})
)
}
return res(
ctx.status(401),
ctx.json({ message: 'Invalid credentials' })
)
})
// Testing token expiration
let requestCount = 0
rest.get('/api/protected-resource', (req, res, ctx) => {
const authHeader = req.headers.get('Authorization')
if (!authHeader || !authHeader.startsWith('Bearer ')) {
return res(
ctx.status(401),
ctx.json({ message: 'Authentication required' })
)
}
// Simulate token expiration after first request
requestCount++
if (requestCount > 1) {
return res(
ctx.status(401),
ctx.json({ message: 'Token expired' })
)
}
return res(
ctx.status(200),
ctx.json({ data: 'Protected content' })
)
})
Organizing MSW Mocks for Large Applications
Large applications require thoughtful organization of mock definitions to maintain testing efficiency and clarity. Structure your mocks by feature, resource type, or API endpoint to create logical groupings that mirror your application architecture. This approach simplifies maintenance and makes finding specific mocks much easier for team members.
Additionally, create reusable response factories or data generators to maintain consistency across different test scenarios. Centralizing mock data creation prevents duplication and ensures tests use realistic, consistent data structures. Subsequently, changes to API contracts require updates in fewer places, making maintenance significantly easier.
// Organization by feature
// src/mocks/features/users/handlers.js
// src/mocks/features/products/handlers.js
// src/mocks/features/orders/handlers.js
// Example of a data factory
// src/mocks/factories/user.js
export function createUser(overrides = {}) {
return {
id: Math.floor(Math.random() * 10000),
username: `user${Math.floor(Math.random() * 1000)}`,
email: `user${Math.floor(Math.random() * 1000)}@example.com`,
createdAt: new Date().toISOString(),
...overrides
}
}
// Using the factory in handlers
import { createUser } from '../factories/user'
rest.get('/api/users/:userId', (req, res, ctx) => {
return res(
ctx.status(200),
ctx.json(createUser({ id: parseInt(req.params.userId) }))
)
})
Debug Strategies for MSW Integration
Debugging MSW setups sometimes presents challenges when unexpected behaviors occur during testing. Enable MSW logging by setting the browser service worker’s options to { onUnhandledRequest: ‘warn’ } to detect unhandled requests that might indicate missing mock definitions. This simple configuration helps identify gaps in your mock coverage.
Furthermore, use browser developer tools to inspect network activity when working with MSW in browser environments. The Network tab clearly shows which requests MSW intercepts and how it responds to them. Therefore, you can quickly identify configuration issues or missing handlers without extensive debugging sessions.
// Enable verbose logging
const worker = setupWorker(...handlers)
worker.start({
onUnhandledRequest: 'warn', // Options: 'bypass', 'warn', 'error'
quiet: false // Set to true in production
})
// Debugging specific handlers
rest.get('/api/troublesome-endpoint', (req, res, ctx) => {
console.log('Request received:', {
url: req.url.toString(),
headers: Object.fromEntries(req.headers.entries()),
params: req.params
})
const response = {
data: 'Test response'
}
console.log('Sending response:', response)
return res(
ctx.status(200),
ctx.json(response)
)
})
Testing Error States and Edge Cases
Testing error states and edge cases reveals potential weaknesses in application error handling. MSW excels at simulating various error responses like validation errors, server errors, or network failures that might be difficult to trigger with real APIs. Your tests can verify that error boundaries, fallback UI, and recovery mechanisms function correctly.
Moreover, testing rate limiting scenarios or partial response failures helps identify resilience issues that might affect users under unusual conditions. These scenarios often reveal edge cases in error handling logic that developers might overlook during normal development. Subsequently, addressing these issues leads to significantly more robust applications.
// Testing various error states
const errorHandlers = [
// 400 Bad Request - Validation Error
rest.post('/api/users', (req, res, ctx) => {
return res(
ctx.status(400),
ctx.json({
errors: {
email: 'Invalid email format',
password: 'Password must be at least 8 characters'
}
})
)
}),
// 404 Not Found
rest.get('/api/users/:userId', (req, res, ctx) => {
return res(
ctx.status(404),
ctx.json({ message: 'User not found' })
)
}),
// 500 Server Error
rest.get('/api/system-status', (req, res, ctx) => {
return res(
ctx.status(500),
ctx.json({ message: 'Internal server error' })
)
}),
// Network failure
rest.get('/api/network-test', (req, res, ctx) => {
return res.networkError('Failed to connect')
})
]
// Use in tests
test('shows appropriate error message when validation fails', async () => {
server.use(...errorHandlers)
// Test implementation
})
MSW for Collaborative Development
MSW facilitates collaborative development between frontend and backend teams by creating a contract-first development approach. Frontend teams can begin implementation immediately after defining API contracts, without waiting for backend implementation completion. This parallel development approach significantly accelerates project timelines and improves team productivity.
Furthermore, MSW mocks serve as living documentation of expected API behavior that both frontend and backend teams can reference. When disagreements arise about expected behavior, the MSW definitions provide a single source of truth for all teams. Therefore, this shared understanding reduces miscommunication and integration problems throughout the development cycle.
Performance Considerations with MSW
Performance considerations matter when implementing MSW in large-scale applications or complex test suites. Minimize the number of handlers loaded simultaneously by organizing them into separate modules and importing only what specific tests need. This approach reduces overhead and improves test execution speed for large test suites.
Additionally, consider conditionally enabling MSW only when needed rather than for all tests. Some tests might not require network interception, and running them without MSW reduces unnecessary overhead. Therefore, selective MSW activation creates more efficient test suites that run faster during continuous integration processes.
// Conditional MSW activation
let server
beforeAll(() => {
// Only start MSW for tests that need it
if (process.env.ENABLE_MSW === 'true') {
server = setupServer(...handlers)
server.listen()
}
})
afterAll(() => {
// Only clean up if server was started
if (server) {
server.close()
}
})
Migrating Existing Tests to MSW
Migrating existing tests to MSW might initially seem daunting but yields significant benefits for test reliability. Start gradually by converting one test suite at a time rather than attempting a complete overhaul. This incremental approach allows your team to adapt to the new testing paradigm without disrupting existing workflows.
Moreover, create a migration plan that prioritizes critical test suites or those with frequent maintenance issues related to mocking. You can temporarily run both old and new approaches in parallel during the transition period. Therefore, this measured approach minimizes risks while steadily improving your testing infrastructure.
Best Practices for RemixPapa.com MSW Integration
Following best practices ensures you get maximum value from your MSW implementation with minimal maintenance overhead. Keep mock responses as close to actual API responses as possible, including all fields and data structures that production endpoints would return. This fidelity prevents subtle bugs caused by differences between test and production environments.
Furthermore, version your mocks alongside your application code to maintain historical accuracy during development. When API contracts change, update mocks immediately to maintain synchronization between frontend expectations and backend reality. Subsequently, this discipline prevents integration issues when deploying updated frontend code with existing backend services.
Future-Proofing Your MSW Setup
Future-proofing your MSW setup requires planning for API changes and evolving testing requirements. Create a systematic process for updating mocks when backend APIs change to maintain testing accuracy over time. This might include automated checks that compare production API responses with mock definitions to detect divergence.
Additionally, consider implementing mock versioning that aligns with your API versioning strategy for testing multiple API versions simultaneously. This capability becomes especially valuable during major API migrations or when supporting multiple client versions. Therefore, thoughtful versioning reduces testing complexity during significant architectural transitions.
Conclusion
RemixPapa.com MSW delivers tremendous value for Remix applications by creating realistic testing environments without complex configuration. The combination of Remix’s data loading patterns with MSW’s request interception creates a perfect testing ecosystem for modern web applications. Your team will benefit from improved testing confidence and accelerated development cycles.
Furthermore, MSW bridges the gap between unit testing and end-to-end testing by providing realistic network simulation without the complexity of maintaining test servers. This middle ground offers the perfect balance of testing fidelity and execution speed. Therefore, RemixPapa.com MSW deserves serious consideration for any Remix project seeking to improve testing practices and application reliability.